Performing MongoDB Atlas CRUD Operations with .NET 6
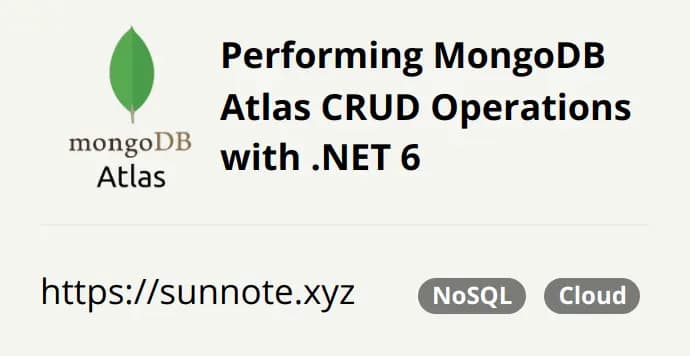
Foreword
πWhile using C# with Microsoft's MSSQL remains the most convenient and user-friendly option, I'm still willing to give it a try and take the opportunity to learn NoSQL. I'll attempt to use C# to perform CRUD operations on MongoDB.
Once you've set up the database and collections in MongoDB Atlas, you can start practicing CRUD operations. You can also directly use the example databases provided by the official documentation for hands-on experience.
You can refer to the previous article for related content. MongoDB Atlas Basic Concepts & Using Compass to Connect MongoDBγ
Database Connection
πOn the official website, under "Database Deployments," click on Connect
> Drivers
, and it will display the connection syntax based on your selected language.
First, you need to install MongoDB.Driver
dotnet add package MongoDB.Driver
Here is the connection string for C#, version 2.13 or later
:
C#const string connectionString = "mongodb+srv://<name>:<password>@cluster0.swvkd15.mongodb.net/?retryWrites=true&w=majority";
Establishing Connection
C#var client = new MongoClient(connectionString);
var collection = client.GetDatabase("sample_analytics").GetCollection<BsonDocument>("customers");
Fetching the customers
collection from the sample_analytics
database allows subsequent operations such as reading and writing to be performed using the collection.
BsonDocument
πBsonDocument
is a data type in MongoDB used to represent documents in the BSON (Binary JSON) format. BSON is a lightweight binary serialization format designed for storing and exchanging data within MongoDB. BsonDocument can be thought of as a dynamic, schema-less document, similar to documents in NoSQL databases.
When storing and exchanging data in MongoDB, it's necessary to convert it to the BsonDocument
format.
Here are some characteristics and uses of BsonDocument:
-
Dynamic Structure: BsonDocument has a dynamic structure that doesn't require defining fields or schemas beforehand, making it highly flexible for handling diverse data.
-
Storing MongoDB Documents: BsonDocument can represent individual documents within MongoDB collections. It contains key-value pairs where keys are field names, and values can be various BSON types (e.g., strings, numbers, dates).
-
Used for Operations: In MongoDB's .NET driver, BsonDocument is often used for executing operations like querying, inserting, updating, and deleting.
-
Serialization Capabilities: BsonDocument can be easily serialized into the BSON format and passed between different applications and languages.
-
Widely Applied: BsonDocument is extensively used in MongoDB development, especially when dealing with dynamic data of varying structures.
Next, is the basic CRUD operation syntax.
Query
πRetrieve All Data
C#var documents = collection.Find(new BsonDocument()).ToList();
Limit Retrieval to the First 10 Records
C#var documents = collection.Find(new BsonDocument()).Limit(10).ToList();
Retrieve Sorted Data, Below is the data retrieved after sorting by the Date
field in descending order.
C#var sort = Builders<BsonDocument>.Sort.Descending("Date");
var sortedDocuments = collection.Find(new BsonDocument()).Sort(sort).ToList();
You can first define the query criteria and then execute the query using the Find
method.
Query all data where the name field is "Alice" and the age field is greater than or equal to 25.
C#var filter = Builders<BsonDocument>.Filter.And(
Builders<BsonDocument>.Filter.Eq("name", "Alice"),
Builders<BsonDocument>.Filter.Gte("age", 25)
);
Query all data where the name field is "Alice" and the age field is either greater than 30 or less than 10.
C#var filter = Builders<BsonDocument>.Filter.And(
Builders<BsonDocument>.Filter.Eq("name", "Alice"),
Builders<BsonDocument>.Filter.Or(
Builders<BsonDocument>.Filter.Gt("age", 30),
Builders<BsonDocument>.Filter.Lt("age", 10)
)
);
Once the query criteria are defined, execute the query using the Find
method.
C#var queryResult = collection.Find(filter).ToList();
Query to Retrieve Specific Parameters
πExample Data:
C#using MongoDB.Bson;
BsonDocument document = new BsonDocument
{
{ "name", "Alice" },
{ "age", 30 },
{ "email", "[email protected]" }
};
Next, I will provide three methods to retrieve values from the document:
- Retrieve Values Using Indexing
C#string name = document["name"].AsString;
int age = document["age"].AsInt32;
string email = document["email"].AsString;
- Retrieve Values Using the GetValue Method, which can prevent situations where the value does not exist
C#BsonValue nameValue;
if (document.TryGetValue("name", out nameValue))
{
string name = nameValue.AsString;
}
- Retrieve Values Using the TryGetElement Method, which allows you to obtain both the key and its corresponding value
C#BsonElement nameElement;
if (document.TryGetElement("name", out nameElement))
{
string name = nameElement.Value.AsString;
}
Convert bsonDocument to List<>
C#List<People> result = documents.Select(bsonDocument => new People
{
name = bsonDocument.GetValue("name").AsString,
age = bsonDocument.GetValue("age").AsInt32,
email = bsonDocument.GetValue("email").AsString,
// Map other properties as needed
}).ToList();
Insert
πJSON-formatted objects can be directly converted into BsonDocument and stored in the database.
C#string jsonString = "{ \"name\": \"Alice\", \"age\": 30, \"email\": \"[email protected]\" }";
BsonDocument document = BsonDocument.Parse(jsonString);
collection.InsertOne(document);
If you want to store multiple records simultaneously, you can use method collection.InsertMany(Multiple);
You can also directly create BsonDocument objects and store them in the database.
Example of Storing Multiple Objects in the Database
C#BsonDocument newDocument1 = new BsonDocument
{
{ "name", "Alice" },
{ "age", 30 }
};
BsonDocument newDocument2 = new BsonDocument
{
{ "name", "Alvin" },
{ "age", 29 }
};
List<BsonDocument> Multiple = new List<BsonDocument>();
Multiple.Add(newDocument1);
Multiple.Add(newDocument2);
collection.InsertMany(Multiple);
Update
πDefine the query criteria to find documents where the name is "Alice".
C#var filter = Builders<BsonDocument>.Filter.Eq("name", "Alice");
Define the operation for updating, where the age field is updated to 31, and the email field is updated to a new value.
C#var update = Builders<BsonDocument>.Update
.Set("age", 31)
.Set("email", "[email protected]");
Use the UpdateOne
method for updating, which will only update the first data that matches the defined criteria.
C#var result = collection.UpdateOne(filter, update);
Use the UpdateMany
method for updating, which will update all data that matches the defined criteria.
C#var result = collection.UpdateMany(filter, update);
Delete
πDeletion, similar to Update, involves first defining the query criteria and then proceeding with the deletion.
Use the DeleteOne
method for deletion, which will delete the first data that matches the defined criteria.
C#var result = collection.DeleteOne(filter);
Use the DeleteMany
method for deletion, which will delete all data that matches the defined criteria.
C#var result = collection.DeleteMany(filter);
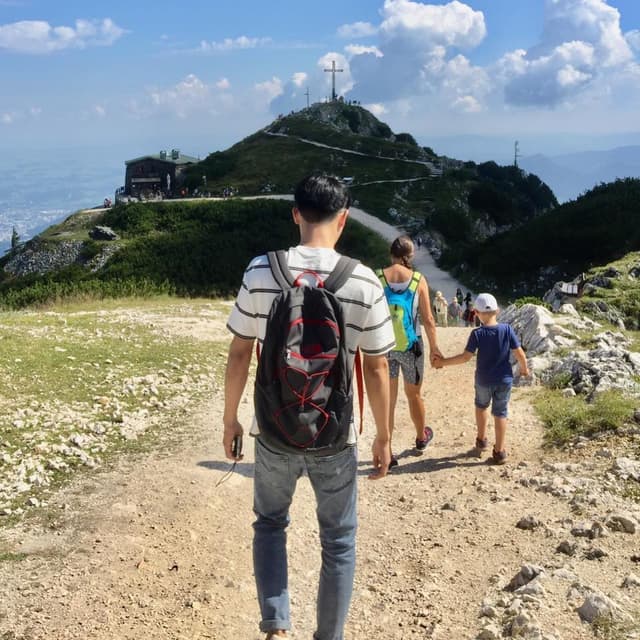
Alvin
Software engineer, interested in financial knowledge, health concepts, psychology, independent travel, and system design.
Related Posts
Discussion (0)
No comments yet.