ASP.NET Core API & Blazor Implements Caching Mechanism to Improve Performance
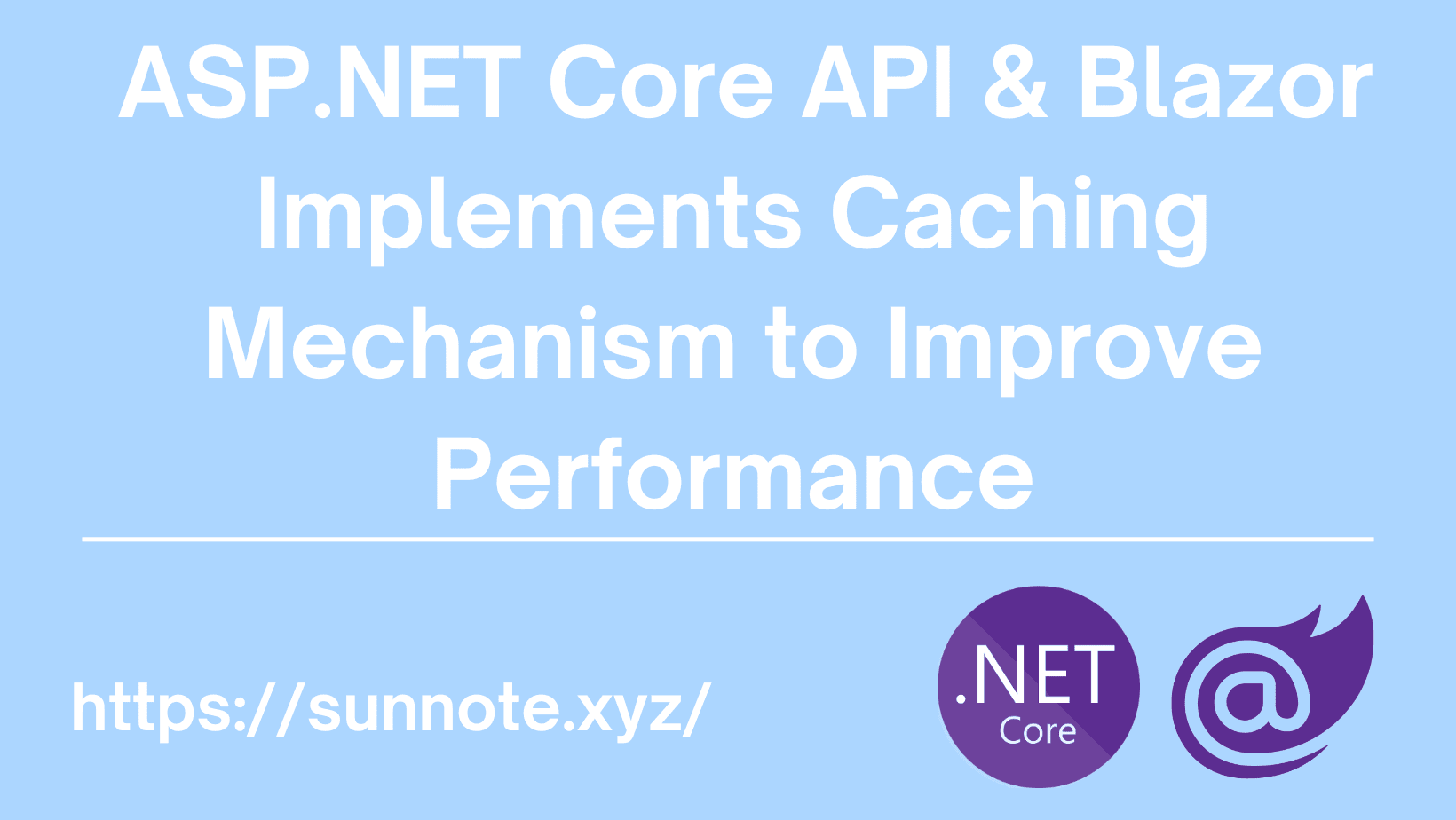
Foreword
🔗In recent projects, due to the large number of thumbnails and calculations, the speed of each screen rendering cannot reach the desired level. In addition to the optimization of the own code and T-SQL, it can also be improved through Lazy loading etc., to achieve the effect of improving data reading speed.
In addition to these methods, caching can also be used to achieve better performance. This article records the use of Blazor WASM architecture and ASP.NET Core API to establish a caching mechanism.
Caching Mechanism
🔗When it comes to caching, it can be understood as using or reading pre-stored data. Because there is no need to re-obtain files from the database or server, the speed can be effectively improved and the load of the website can be reduced.
If the website is a static website, or there is a need for a large number of static resources, if every time a user enters the website, the resources need to be loaded, there will inevitably be a fluency problem. In this case, if you use a CDN (Content Delivery Network) service , through a network composed of server nodes distributed around the world, the transmission speed and performance of static resources can be effectively improved. Caching technology is used to achieve efficient transmission by pre-stored static resources in various places.
Within the backend API, a similar caching mechanism can be implemented to facilitate efficient data transmission by pre-storing data in memory. This article aims to introduce the usage of the MemoryCache
class for caching purposes. For larger websites or distributed systems, the IDistributedCache
class can be employed; however, this might require me additional time for understanding and implementation
Appropriate usage situations
🔗If you want to use the MemoryCache
class for caching, since the data is stored in memory, if the application is closed or restarted, the system fails, or the system itself manages and optimizes the memory, the stored data will disappear. Therefore, caching is not recommended to store important data, or data that changes frequently, resulting in incorrect website content information.
Taking this article as an example, the project website will display a cover image on the homepage. Since the cover image is not updated frequently, and the data has been stored in the database and AWS S3, it is a good way to pre-store it in the cache to improve the speed at which users load the homepage.
Code
🔗Create cache
🔗The architecture, whether it is Blazor WASM or general Web API, is to modify the API in the controller.
code show as below:
C#using Microsoft.Extensions.Caching.Memory;
namespace BlazorApp.Server.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class StorageController : ControllerBase
{
private readonly IStorageService _storageService;
private readonly IMemoryCache _cache;
public StorageController(IStorageService storageService, IMemoryCache memoryCache)
{
_storageService = storageService;
_cache = memoryCache;
}
[HttpGet("logo/admin-no-cache")]
public async Task<List<S3FileInfo>> GetLogoFileNoCache()
{
List<S3FileInfo> FileInfo = await _storageService.GetFileByKeyAsync();
return FileInfo;
}
[HttpGet("logo")]
public async Task<List<S3FileInfo>> GetLogoFile()
{
string cacheKey = "logo";
if (!_cache.TryGetValue<List<S3FileInfo>>(cacheKey, out List<S3FileInfo> cachedData))
{
List<S3FileInfo> FileInfo = await _storageService.GetFileByKeyAsync();
cachedData = FileInfo;
_cache.Set(cacheKey, cachedData, TimeSpan.FromHours(24));
}
return cachedData;
}
}
}
There are two APIs, one is logo/admin-no-cache
, which is used by administrators. Administrators need to confirm the correctness of the data immediately, and it is a no-cache version.
The other one is logo
, which is used when loading the home page. The logic is to set the cache Key to logo
. Every time you call, you need to confirm whether the cache contains data with the key logo
.
If it exists, use it; if not, retrieve the data.
_cache.Set(cacheKey, cachedData, TimeSpan.FromHours(24));
After obtaining the data, store the data in the cache and set it to expire in 24 hours. This means that every 24 hours, the cache will disappear, and the loading speed of the first person who reads the website will return to normal because the data must be retrieve again, and every subsequent user will still be just as efficient and fast. 😎
Remove cache
🔗Sometimes there is a need to clear the cache, such as clearing the cache after data is updated to allow users to see real-time content.
Remove specific cache codes:
C#_cache.Remove("logo");
Get cache list
🔗After create cache feature, it is necessary to provide admin with the ability to remove the cache for frequently update info. They can obtain the cache key list currently stored in the memory and then further delete it with above Remove cache method.
C#[HttpGet("cache")]
public async Task<List<string>> GetCacheList()
{
var field = typeof(MemoryCache).GetProperty("EntriesCollection", BindingFlags.NonPublic | BindingFlags.Instance);
var collection = field.GetValue(_cache) as ICollection;
var items = new List<string>();
if (collection != null)
foreach (var item in collection)
{
var methodInfo = item.GetType().GetProperty("Key");
var val = methodInfo.GetValue(item);
items.Add(val.ToString());
}
return items;
}
Conclusion
🔗The above is how to use ASP.NET Core to implement the memory caching mechanism. Personally, I divide the data that needs to be cached into two APIs, which is easier to classify. In fact, you can determine whether to retrieve cached or actual data by modifying the API conditions. If the website uses a cloud database or S3 and other services, if it can make good use of cache, I believe it can reduce a lot of data transmission costs.😎
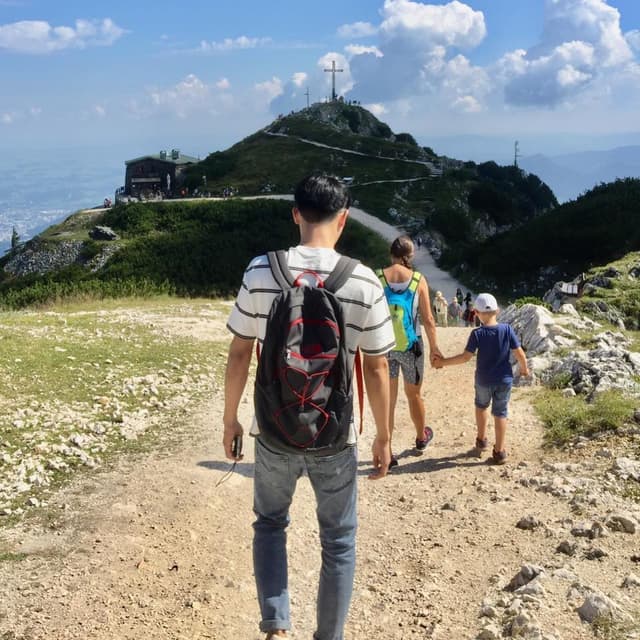
Alvin
Software engineer, interested in financial knowledge, health concepts, psychology, independent travel, and system design.
Related Posts
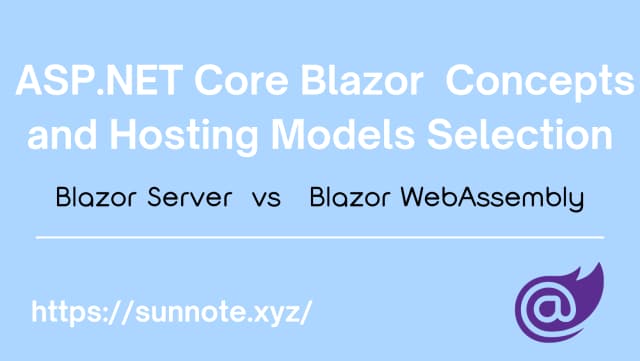
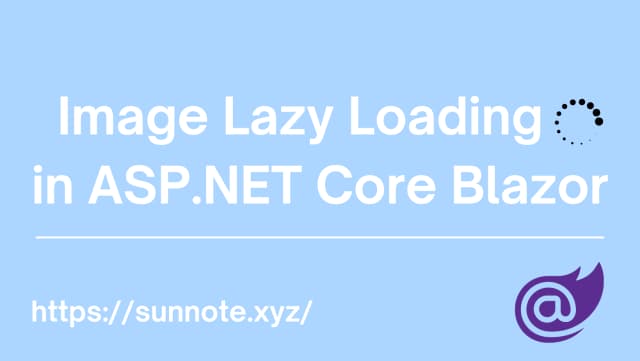
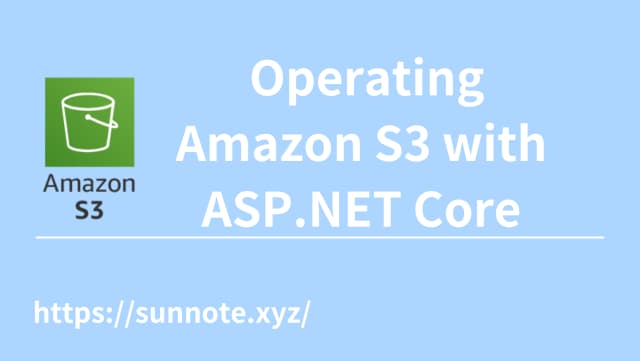
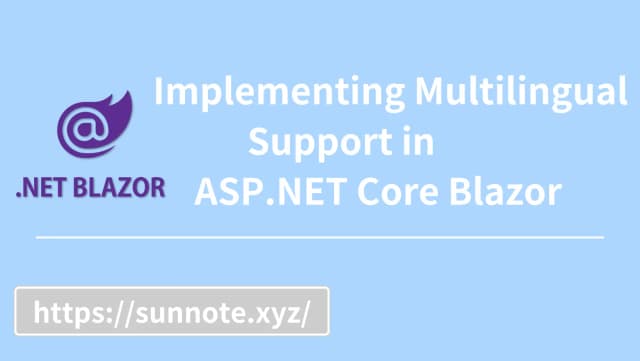
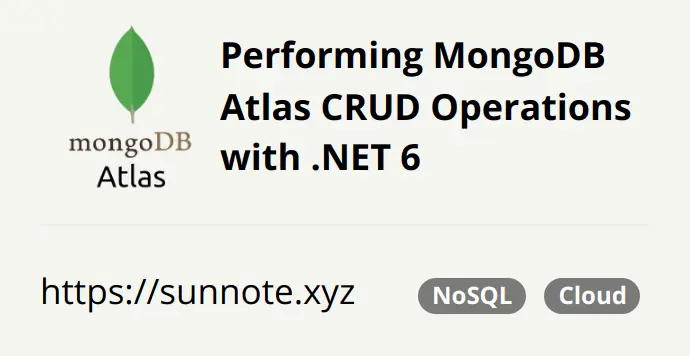
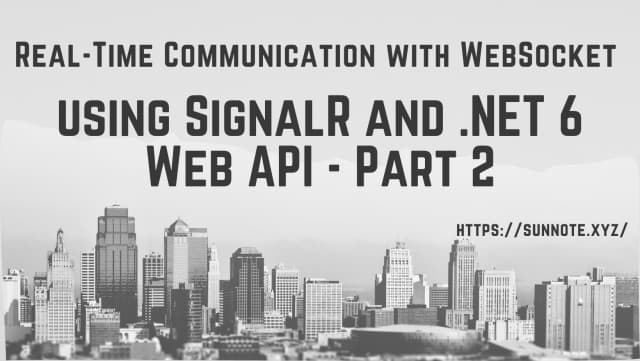