使用 .NET 6 進行 MongoDB Atlas CRUD 操作
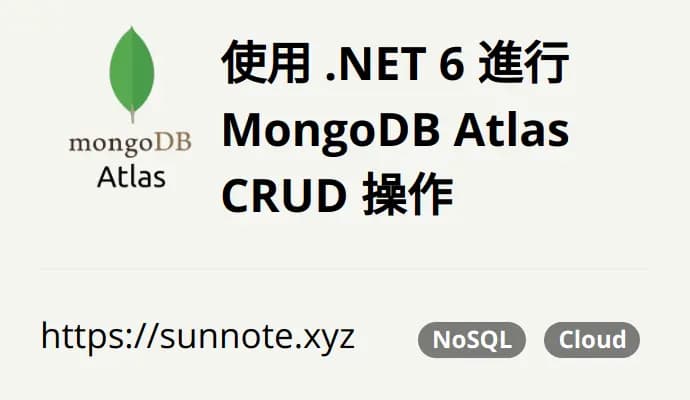
前言
🔗雖然使用 C# 還是本身微軟的 MSSQL 最方便好用,但還是試著嘗試藉由學習 NoSQL 這個機會,來嘗試使用 C# 操作 MongoDB 的 CRUD。
使用 MongoDB Atlas 建立好 Database 還有collection 後,就可以進行 CRUD 的練習,也可以直接使用官方給的範例資料庫。
相關內容可以查詢上一篇 MongoDB Atlas 資料庫基礎觀念、操作流程與使用 Compass 連線。
資料庫連線
🔗在官網的Database Deployments 點選 Connect
> Drivers
就會顯示依據選擇的語言的連線語法。
首先需為專案安裝 MongoDB.Driver
dotnet add package MongoDB.Driver
以下為 C#,版本 2.13 or later
的連線字串
C#const string connectionString = "mongodb+srv://<name>:<password>@cluster0.swvkd15.mongodb.net/?retryWrites=true&w=majority";
建立連線
C#var client = new MongoClient(connectionString);
var collection = client.GetDatabase("sample_analytics").GetCollection<BsonDocument>("customers");
取得sample_analytics
中的customers
集合,後續可藉由collection進行相關讀寫操作。
BsonDocument
🔗BsonDocument
是 MongoDB 中的一種資料類型,用於表示 BSON(Binary JSON)格式的文件。BSON 是一種輕量級的二進制序列化格式,用於在 MongoDB 中儲存和交換資料。BsonDocument
可以看作是一個動態的、無模式的文件,類似於 NoSQL 資料庫中的文檔。
在 MongoDB 中進行儲存和交換資料時都需要先轉換成BsonDocument
的格式。
以下是BsonDocument
的一些特點和用途:
-
動態結構: BsonDocument 是動態結構的,不需要事先定義欄位或模式,這使得它在處理多樣性資料時非常靈活。
-
儲存 MongoDB 文件: BsonDocument 可以代表 MongoDB 集合中的單個文件。它包含了鍵值對,其中鍵是字段名,值可以是各種 BSON 類型(如字符串、數字、日期等)。
-
用於操作: 在 MongoDB 的 .NET 驅動程式中,BsonDocument 通常用於執行查詢、插入、更新和刪除等操作。
-
可序列化: BsonDocument 可以輕鬆地序列化成 BSON 格式,並且可以在不同應用程式和語言之間進行傳遞。
-
應用廣泛: BsonDocument 在 MongoDB 開發中被廣泛使用,特別是在需要處理不同結構的動態資料時。
接下來紀錄一下CRUD的基本操作語法。
查詢
🔗取得所有的資料
C#var documents = collection.Find(new BsonDocument()).ToList();
限制取得前10筆資料
C#var documents = collection.Find(new BsonDocument()).Limit(10).ToList();
取得排序後的資料,以下為取得按Date
欄位降序排序後的資料。
C#var sort = Builders<BsonDocument>.Sort.Descending("Date");
var sortedDocuments = collection.Find(new BsonDocument()).Sort(sort).ToList();
可以先定義查詢條件再藉由Find
方法執行查詢
查詢 name 欄位為 "Alice" 且 age 欄位大於等於 25 的所有資料
C#var filter = Builders<BsonDocument>.Filter.And(
Builders<BsonDocument>.Filter.Eq("name", "Alice"),
Builders<BsonDocument>.Filter.Gte("age", 25)
);
查詢 name 欄位為 "Alice" 且 (age > 30 或 age < 10) 的所有資料
C#var filter = Builders<BsonDocument>.Filter.And(
Builders<BsonDocument>.Filter.Eq("name", "Alice"),
Builders<BsonDocument>.Filter.Or(
Builders<BsonDocument>.Filter.Gt("age", 30),
Builders<BsonDocument>.Filter.Lt("age", 10)
)
);
定義好查詢條件後,再使用Find
方法執行查詢
C#var queryResult = collection.Find(filter).ToList();
查詢取得特定參數
🔗範例資料:
C#using MongoDB.Bson;
BsonDocument document = new BsonDocument
{
{ "name", "Alice" },
{ "age", 30 },
{ "email", "[email protected]" }
};
接下來提供3種方法取得document
的值:
- 使用索引獲取值
C#string name = document["name"].AsString;
int age = document["age"].AsInt32;
string email = document["email"].AsString;
- 使用 GetValue 方法獲取值,此方法可以預防值不存在的情況
C#BsonValue nameValue;
if (document.TryGetValue("name", out nameValue))
{
string name = nameValue.AsString;
}
- 使用 TryGetElement 方法獲取值,可以取得鍵與對應的值
C#BsonElement nameElement;
if (document.TryGetElement("name", out nameElement))
{
string name = nameElement.Value.AsString;
}
將 bsonDocument 轉為 List<>
C#List<People> result = documents.Select(bsonDocument => new People
{
name = bsonDocument.GetValue("name").AsString,
age = bsonDocument.GetValue("age").AsInt32,
email = bsonDocument.GetValue("email").AsString,
// Map other properties as needed
}).ToList();
新增
🔗Json格式的物件,可以直接轉換成BsonDocument即可存入DB
C#string jsonString = "{ \"name\": \"Alice\", \"age\": 30, \"email\": \"[email protected]\" }";
BsonDocument document = BsonDocument.Parse(jsonString);
collection.InsertOne(document);
若要同時存入多筆則可使用collection.InsertMany(Multiple);
也可以直接建立BsonDocument物件再存入DB
範例為儲存多個物件至DB
C#BsonDocument newDocument1 = new BsonDocument
{
{ "name", "Alice" },
{ "age", 30 }
};
BsonDocument newDocument2 = new BsonDocument
{
{ "name", "Alvin" },
{ "age", 29 }
};
List<BsonDocument> Multiple = new List<BsonDocument>();
Multiple.Add(newDocument1);
Multiple.Add(newDocument2);
// 插入BsonDocument到集合中
collection.InsertMany(Multiple);
編輯
🔗定義要查詢的條件,找到 name 為 "Alice" 的文件。
C#var filter = Builders<BsonDocument>.Filter.Eq("name", "Alice");
定義要進行更新的操作,將 age 欄位更新為 31,email 欄位更新為新的值。
C#var update = Builders<BsonDocument>.Update
.Set("age", 31)
.Set("email", "[email protected]");
使用 UpdateOne
方法進行更新,只會更新符合定義的第一筆資料。
C#var result = collection.UpdateOne(filter, update);
使用 UpdateMany
方法進行更新,更新符合定義的所有資料。
C#var result = collection.UpdateMany(filter, update);
刪除
🔗刪除與編輯相同,都是先定義查詢的條件,再進行刪除。
使用 DeleteOne
方法進行刪除,刪除符合條件的第一筆資料。
C#var result = collection.DeleteOne(filter);
使用 DeleteMany
方法進行刪除,刪除符合條件的所有資料。
C#var result = collection.DeleteMany(filter);
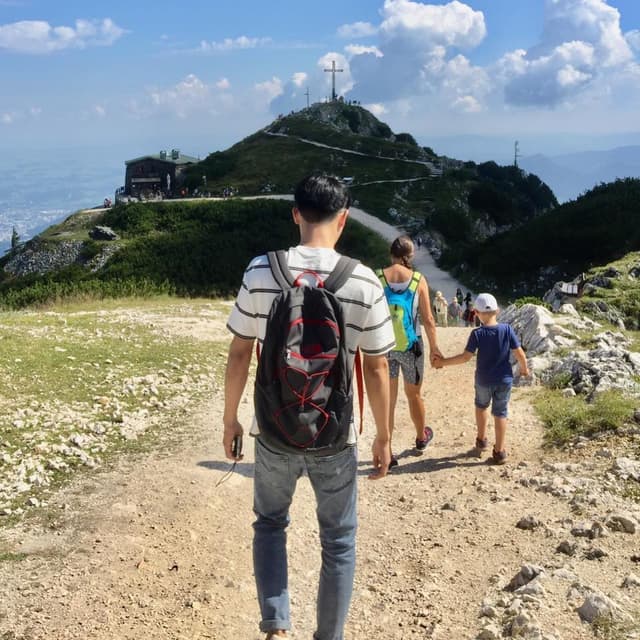
Alvin
軟體工程師,喜歡金融知識、健康觀念、心理哲學、自助旅遊與系統設計。
相關文章
留言區 (0)
尚無留言